Signed URLs
Secure URLs of images by adding security token to URLs
Securing image URLs can add an extra layer of security. It helps to prevent unauthorized access to your image URL.
We use an 'MD5' hash function on the original URL and a secure token to generate the signature. This signature needs to pass with an unsigned URL with the parameter s
to make a signed URL. If the URL is altered or missing the s
parameter, we will respond with 403 - Forbidden
status.
Enable Secure URLs
By default, this feature is disabled for any source. You can enable it by following the steps.
- Go to image sources page, and edit the source to enable a secure URL.
- Click on the security tab.
- Toggle the 'Secure URLs' button and click Save.
Your secure token will appear below this option to use it to sign a URL.
Please read this
Enabling secure URLs for existing source will lead to 403 errors on all URLs unless all requests are signed. Please enable at your own discretion and with appropriate knowledge.
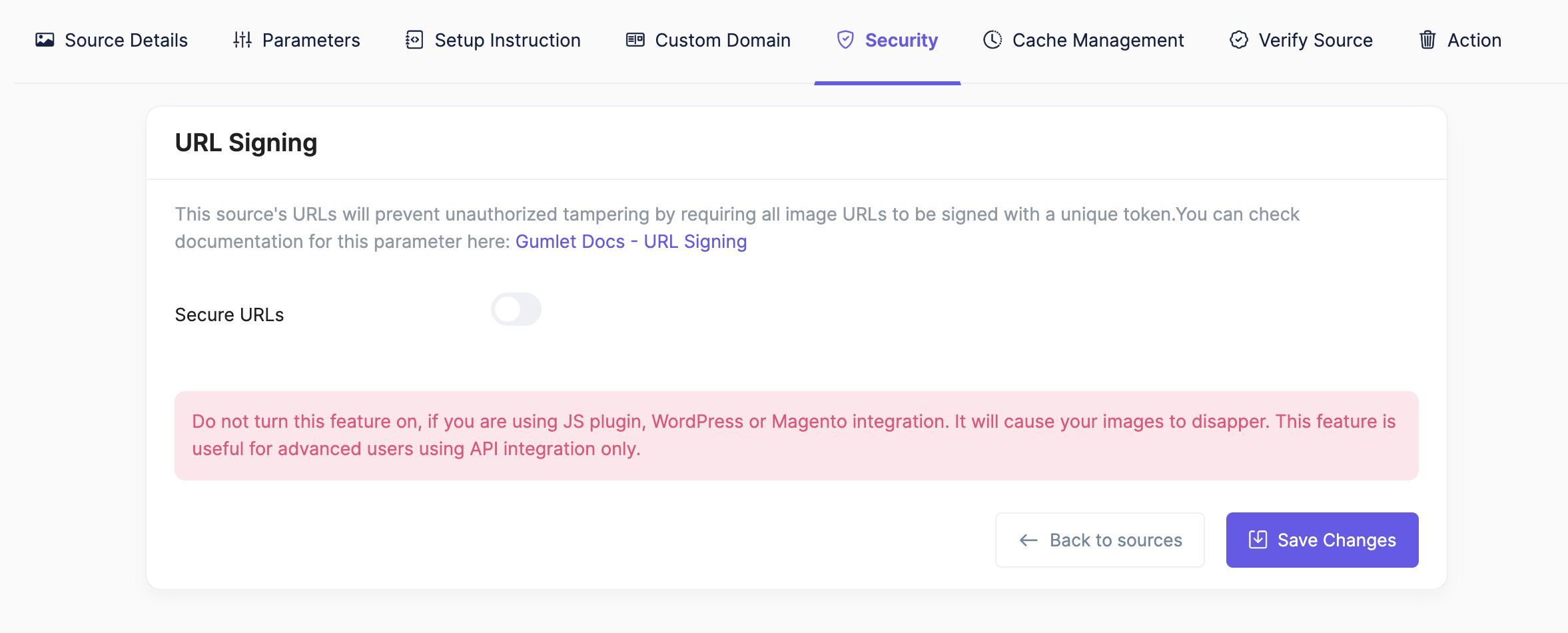
Signing URLs
We use 'MD5' a cryptographic hash function to sign the image URL. Your secure token, image URL, and query parameters will be input to this function. The output of this hash function will be appended to the end of your unsigned URL with thes
parameter.
Here is the sample Nodejs code to sign any URL.
const crypto = require("crypto");
const gumlet_source = 'demo.gumlet.com';
const image_path = 'fell.jpeg';
const query_params = 'width=300'
const signed_secret = 'sample123xyz';
const unsigned_url = signed_secret + '/' + image_path + '?' + query_params;
const hash = crypto.createHash('md5').update(unsigned_url).digest('hex');
const signed_url = 'https://' + gumlet_source + '/' + image_path + '?' + query_params + '&s=' + hash;
console.log(signed_url);
//->> https://demo.gumlet.io/fall.jpeg?width=300&s=xxxxxxxxxxxxxxxxxxxx
You can reference this code and implement URL signing in the language of your choice. We will soon publish examples of URL signing in different languages.
Expiry
URLs can be given an expiration date via an expires
parameter that takes a UNIX timestamp in the query parameters like ?expires=1584199888
Any request after this timestamp will be 403 - expired. We set the remaining expiration time as max-age in the cache-control header for valid requests
Updated 10 months ago