Audio Transcoding
Reference for audio-only transcoding and delivery.
Gumlet allows you to transcode and deliver only audio streams via CMS or APIs. Audio streams are streamed through adaptive bitrate streaming formats like HLS
and DASH
, also it can be delivered in MP4
format.
via CMS
You can upload the audio files in a similar way you upload videos. Visit the dashboard and click on the Upload button in the bottom-right corner.
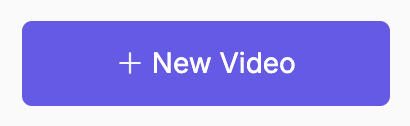
via API
To process audio-only assets, you need to send the audio_only
flag in Create Asset API, and provide an audio file in any of the following formats: mp3
, opus
, flac
, wav
, ac3
, m4a
, aac
.
curl -L -X POST 'https://api.gumlet.com/v1/video/assets' \
-H 'Authorization: Bearer <YOUR API KEY>' \
-H 'Content-Type: application/json' \
--data-raw '{
"input":"https://gumlet.sgp1.digitaloceanspaces.com/video/sample_1.aac",
"source_id": "5f462c1561cf8a766464ffc4",
"format": "hls",
"audio_only": true
}'
{
"asset_id": "61973239b4670bdc996729d5",
"progress": 0,
"created_at": 1637298745987,
"status": "queued",
"tag": [],
"source_id": "5f462c1561cf8a766464ffc4",
"input": {
"transformations": {
"format": "hls",
"audio_codec": [
"aac"
],
"video_codec": [
"libx264"
],
"thumbnail": [
"auto"
],
"thumbnail_format": "png",
"mp4_access": false,
"audio_only": true,
"keep_original": true,
"per_title_encoding": true,
"process_low_resolution_input": false
},
"source_url": "https://gumlet.sgp1.digitaloceanspaces.com/video/sample_1.aac",
"additional_tracks": []
},
"output": {
"format": "hls",
"status_url": "https://api.gumlet.com/v1/video/assets/61973239b4670bdc996729d5",
"playback_url": "https://video.gumlet.io/5f462c1561cf8a766464ffc4/61973239b4670bdc996729d5/1.m3u8"
}
}
Updated 2 months ago